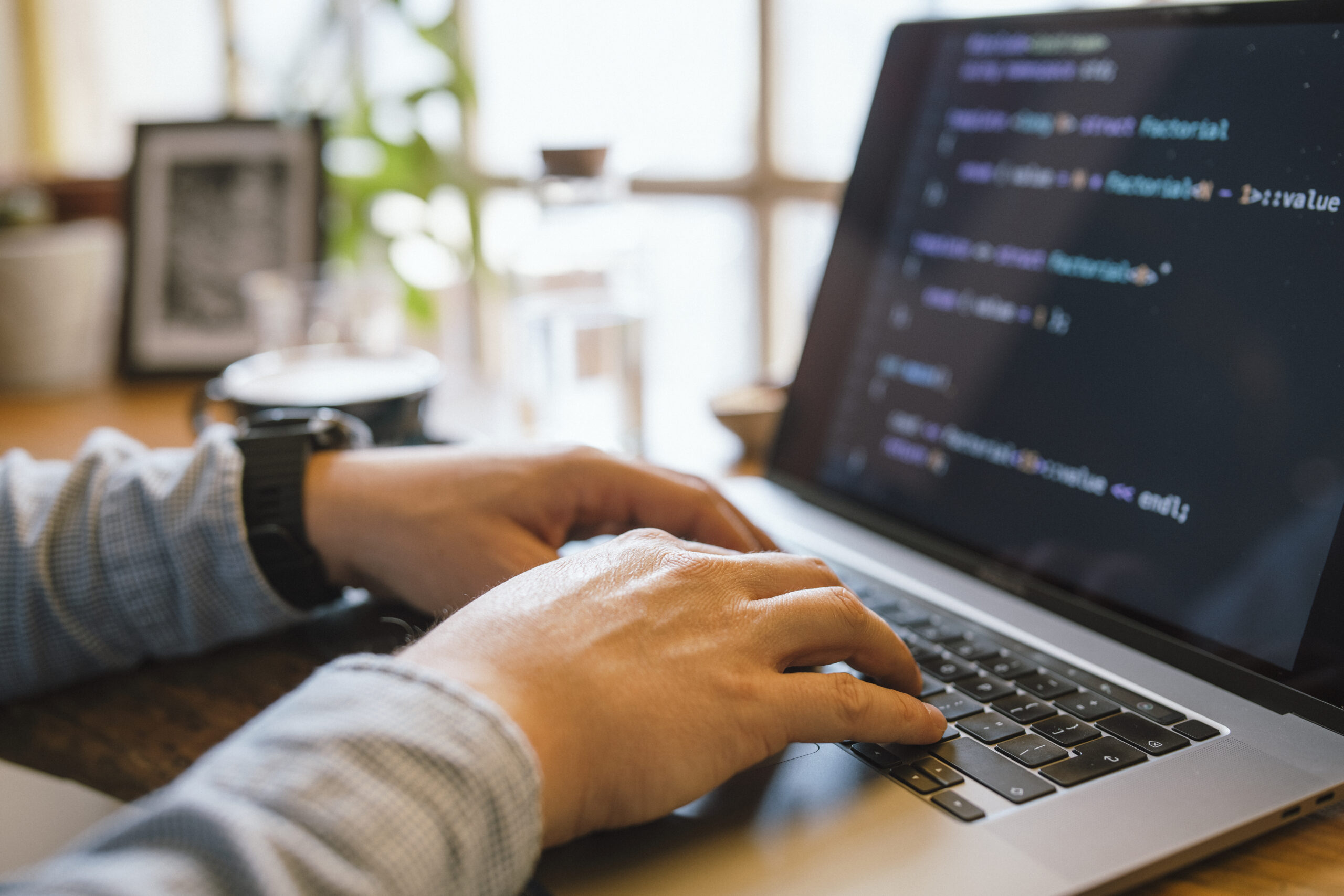
Debugging is Just about the most vital — nonetheless frequently disregarded — techniques inside of a developer’s toolkit. It isn't nearly repairing broken code; it’s about comprehension how and why points go Completely wrong, and learning to Consider methodically to resolve troubles successfully. Irrespective of whether you are a starter or simply a seasoned developer, sharpening your debugging competencies can help you save several hours of irritation and radically help your efficiency. Here's various tactics that can help builders degree up their debugging sport by me, Gustavo Woltmann.
Master Your Applications
On the list of fastest approaches developers can elevate their debugging expertise is by mastering the applications they use every day. While crafting code is just one Section of advancement, realizing how you can connect with it proficiently for the duration of execution is equally vital. Modern-day advancement environments come Geared up with effective debugging capabilities — but quite a few developers only scratch the area of what these applications can perform.
Just take, as an example, an Built-in Enhancement Setting (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These resources allow you to established breakpoints, inspect the value of variables at runtime, action via code line by line, and perhaps modify code over the fly. When utilised properly, they Enable you to observe particularly how your code behaves throughout execution, that is a must have for tracking down elusive bugs.
Browser developer instruments, like Chrome DevTools, are indispensable for entrance-end developers. They permit you to inspect the DOM, watch network requests, look at genuine-time effectiveness metrics, and debug JavaScript within the browser. Mastering the console, resources, and network tabs can convert frustrating UI troubles into workable tasks.
For backend or technique-level developers, applications like GDB (GNU Debugger), Valgrind, or LLDB offer deep Management around operating procedures and memory management. Understanding these instruments may have a steeper Studying curve but pays off when debugging effectiveness issues, memory leaks, or segmentation faults.
Outside of your IDE or debugger, become comfy with Edition Management systems like Git to comprehend code history, discover the exact second bugs have been launched, and isolate problematic improvements.
Ultimately, mastering your equipment suggests likely further than default configurations and shortcuts — it’s about developing an intimate knowledge of your progress natural environment so that when issues arise, you’re not lost in the dark. The better you recognize your equipment, the more time you'll be able to shell out fixing the actual difficulty rather then fumbling as a result of the procedure.
Reproduce the situation
Among the most important — and sometimes missed — ways in helpful debugging is reproducing the situation. In advance of jumping in to the code or making guesses, developers need to produce a reliable setting or situation exactly where the bug reliably seems. Without reproducibility, correcting a bug gets a recreation of chance, often bringing about wasted time and fragile code adjustments.
The first step in reproducing a dilemma is collecting as much context as is possible. Request questions like: What steps brought about the issue? Which ecosystem was it in — growth, staging, or production? Are there any logs, screenshots, or mistake messages? The more depth you may have, the less difficult it becomes to isolate the precise conditions underneath which the bug occurs.
When you finally’ve gathered sufficient facts, attempt to recreate the problem in your neighborhood atmosphere. This may necessarily mean inputting precisely the same data, simulating very similar user interactions, or mimicking technique states. If the issue appears intermittently, take into account writing automated checks that replicate the edge scenarios or state transitions concerned. These checks not simply assist expose the situation but also avert regressions Down the road.
Occasionally, The problem could be natural environment-specific — it would materialize only on particular working devices, browsers, or below distinct configurations. Applying tools like virtual devices, containerization (e.g., Docker), or cross-browser screening platforms is often instrumental in replicating such bugs.
Reproducing the situation isn’t simply a step — it’s a state of mind. It calls for endurance, observation, in addition to a methodical solution. But when you finally can continuously recreate the bug, you might be already halfway to fixing it. Having a reproducible situation, You can utilize your debugging applications more effectively, test prospective fixes securely, and talk a lot more Obviously with all your workforce or buyers. It turns an summary grievance into a concrete challenge — and that’s where developers thrive.
Read and Understand the Mistake Messages
Mistake messages in many cases are the most useful clues a developer has when a little something goes Completely wrong. In lieu of observing them as annoying interruptions, developers ought to discover to take care of error messages as immediate communications with the technique. They generally let you know precisely what happened, where by it happened, and in some cases even why it took place — if you understand how to interpret them.
Start by examining the concept cautiously As well as in full. Quite a few developers, especially when underneath time stress, look at the primary line and right away commence making assumptions. But further within the mistake stack or logs could lie the true root bring about. Don’t just copy and paste error messages into search engines — read through and comprehend them first.
Split the mistake down into elements. Can it be a syntax mistake, a runtime exception, or perhaps a logic mistake? Does it position to a specific file and line variety? What module or function activated it? These questions can guideline your investigation and level you towards the responsible code.
It’s also valuable to understand the terminology on the programming language or framework you’re using. Error messages in languages like Python, JavaScript, or Java generally adhere to predictable designs, and learning to recognize these can considerably speed up your debugging approach.
Some faults are obscure or generic, As well as in These situations, it’s essential to examine the context where the mistake occurred. Examine associated log entries, input values, and up to date variations within the codebase.
Don’t forget about compiler or linter warnings both. These normally precede bigger challenges and supply hints about possible bugs.
Eventually, mistake messages are certainly not your enemies—they’re your guides. Learning to interpret them properly turns chaos into clarity, aiding you pinpoint troubles quicker, minimize debugging time, and become a far more successful and self-confident developer.
Use Logging Sensibly
Logging is One of the more powerful equipment in the developer’s debugging toolkit. When applied correctly, it offers real-time insights into how an software behaves, helping you understand what’s happening under the hood with no need to pause execution or stage with the code line by line.
A great logging technique starts with understanding what to log and at what level. Common logging amounts contain DEBUG, Information, WARN, Mistake, and Lethal. Use DEBUG for specific diagnostic data for the duration of advancement, Facts for normal functions (like profitable commence-ups), WARN for potential challenges that don’t split the appliance, ERROR for real problems, and Lethal in the event the technique check here can’t proceed.
Steer clear of flooding your logs with excessive or irrelevant facts. Too much logging can obscure significant messages and slow down your method. Deal with critical activities, point out improvements, input/output values, and critical conclusion factors inside your code.
Structure your log messages Obviously and continuously. Incorporate context, like timestamps, ask for IDs, and function names, so it’s easier to trace difficulties in distributed devices or multi-threaded environments. Structured logging (e.g., JSON logs) might make it even much easier to parse and filter logs programmatically.
In the course of debugging, logs Permit you to monitor how variables evolve, what conditions are achieved, and what branches of logic are executed—all with no halting the program. They’re Specially useful in output environments in which stepping as a result of code isn’t achievable.
On top of that, use logging frameworks and applications (like Log4j, Winston, or Python’s logging module) that help log rotation, filtering, and integration with checking dashboards.
Ultimately, smart logging is about equilibrium and clarity. Having a very well-thought-out logging strategy, you could decrease the time it takes to spot troubles, attain deeper visibility into your programs, and Increase the overall maintainability and reliability of the code.
Believe Just like a Detective
Debugging is not simply a technological job—it's a kind of investigation. To correctly identify and resolve bugs, builders must method the method similar to a detective resolving a secret. This mindset assists break down sophisticated issues into manageable components and stick to clues logically to uncover the basis bring about.
Get started by accumulating evidence. Look at the signs and symptoms of the situation: mistake messages, incorrect output, or general performance issues. Just like a detective surveys a crime scene, collect as much relevant info as you are able to with out leaping to conclusions. Use logs, take a look at scenarios, and consumer studies to piece collectively a clear image of what’s happening.
Subsequent, form hypotheses. Question by yourself: What may be triggering this habits? Have any adjustments just lately been manufactured on the codebase? Has this concern occurred right before underneath very similar situation? The target is usually to slim down prospects and determine possible culprits.
Then, test your theories systematically. Attempt to recreate the problem in a managed setting. Should you suspect a specific functionality or ingredient, isolate it and confirm if the issue persists. Similar to a detective conducting interviews, inquire your code questions and Enable the final results lead you nearer to the truth.
Pay near interest to compact aspects. Bugs generally conceal in the the very least anticipated places—similar to a missing semicolon, an off-by-just one error, or simply a race situation. Be extensive and affected person, resisting the urge to patch The difficulty without having absolutely comprehension it. Non permanent fixes may possibly disguise the real challenge, just for it to resurface later.
And finally, continue to keep notes on Whatever you tried using and realized. Equally as detectives log their investigations, documenting your debugging procedure can preserve time for upcoming concerns and help Other folks understand your reasoning.
By pondering just like a detective, builders can sharpen their analytical abilities, technique complications methodically, and turn out to be simpler at uncovering concealed issues in advanced systems.
Compose Assessments
Crafting checks is one of the most effective strategies to transform your debugging skills and General enhancement performance. Tests not just aid catch bugs early but in addition function a security Internet that offers you assurance when making improvements towards your codebase. A perfectly-analyzed software is much easier to debug mainly because it allows you to pinpoint precisely exactly where and when an issue occurs.
Start with device assessments, which target specific features or modules. These modest, isolated assessments can speedily reveal no matter whether a particular piece of logic is Operating as predicted. Every time a take a look at fails, you quickly know the place to seem, drastically minimizing time invested debugging. Unit checks are Primarily useful for catching regression bugs—issues that reappear following previously being preset.
Upcoming, integrate integration tests and end-to-close assessments into your workflow. These aid make sure that different parts of your software get the job done collectively smoothly. They’re significantly handy for catching bugs that take place in complicated systems with various parts or companies interacting. If one thing breaks, your tests can inform you which A part of the pipeline unsuccessful and beneath what conditions.
Producing exams also forces you to definitely Feel critically regarding your code. To check a element appropriately, you'll need to be familiar with its inputs, anticipated outputs, and edge conditions. This amount of understanding In a natural way leads to higher code structure and less bugs.
When debugging a difficulty, creating a failing check that reproduces the bug is often a powerful initial step. As soon as the examination fails continuously, you'll be able to center on fixing the bug and observe your exam pass when The problem is settled. This tactic ensures that the identical bug doesn’t return Down the road.
In brief, producing checks turns debugging from a annoying guessing activity into a structured and predictable method—serving to you capture far more bugs, a lot quicker and much more reliably.
Just take Breaks
When debugging a tough difficulty, it’s simple to become immersed in the trouble—observing your monitor for several hours, trying Resolution immediately after Remedy. But The most underrated debugging instruments is actually stepping absent. Getting breaks allows you reset your intellect, reduce aggravation, and often see the issue from a new perspective.
When you're way too near to the code for way too prolonged, cognitive tiredness sets in. You would possibly start out overlooking evident glitches or misreading code you wrote just hrs previously. On this condition, your brain becomes considerably less productive at difficulty-solving. A short wander, a espresso split, or perhaps switching to a different task for ten–quarter-hour can refresh your target. Several developers report locating the root of a problem when they've taken time and energy to disconnect, allowing their subconscious function in the history.
Breaks also enable avert burnout, Specifically throughout for a longer period debugging classes. Sitting in front of a screen, mentally trapped, is not merely unproductive but additionally draining. Stepping absent lets you return with renewed Power and a clearer way of thinking. You could suddenly detect a missing semicolon, a logic flaw, or simply a misplaced variable that eluded you ahead of.
In the event you’re trapped, a fantastic rule of thumb should be to set a timer—debug actively for forty five–60 minutes, then have a 5–ten moment split. Use that point to move all-around, stretch, or do a little something unrelated to code. It might really feel counterintuitive, Primarily below limited deadlines, nevertheless it basically contributes to a lot quicker and more effective debugging In the long term.
In brief, getting breaks is not a sign of weak spot—it’s a smart approach. It presents your brain House to breathe, improves your point of view, and allows you avoid the tunnel eyesight That always blocks your progress. Debugging is actually a psychological puzzle, and relaxation is part of fixing it.
Master From Each and every Bug
Each individual bug you experience is a lot more than simply a temporary setback—It truly is a possibility to grow as being a developer. No matter if it’s a syntax mistake, a logic flaw, or a deep architectural concern, each can train you a little something beneficial should you make the effort to replicate and evaluate what went Mistaken.
Start out by inquiring yourself a couple of crucial inquiries as soon as the bug is fixed: What prompted it? Why did it go unnoticed? Could it happen to be caught earlier with much better procedures like unit screening, code evaluations, or logging? The solutions normally expose blind places as part of your workflow or being familiar with and assist you Establish much better coding patterns going ahead.
Documenting bugs can even be a wonderful pattern. Continue to keep a developer journal or manage a log in which you Notice down bugs you’ve encountered, how you solved them, and Everything you uncovered. After a while, you’ll start to see patterns—recurring issues or popular issues—you can proactively prevent.
In crew environments, sharing Everything you've discovered from the bug with the peers may be Particularly powerful. Whether it’s via a Slack concept, a short write-up, or A fast information-sharing session, assisting others steer clear of the identical issue boosts staff effectiveness and cultivates a stronger Mastering tradition.
More importantly, viewing bugs as classes shifts your state of mind from irritation to curiosity. As an alternative to dreading bugs, you’ll begin appreciating them as critical aspects of your advancement journey. After all, many of the greatest builders usually are not those who create fantastic code, but people who consistently find out from their issues.
Ultimately, Each individual bug you resolve provides a brand new layer on your skill set. So subsequent time you squash a bug, have a instant to reflect—you’ll appear absent a smarter, much more able developer because of it.
Conclusion
Increasing your debugging skills will take time, observe, and patience — even so the payoff is large. It makes you a more successful, self-assured, and capable developer. The following time you happen to be knee-deep inside a mysterious bug, don't forget: debugging isn’t a chore — it’s a possibility to be much better at Whatever you do.